Introduction
The Trust.med API Allows you to gather and edit multiple different facets of data within your Trust.med account.
As a manufacturer, you will be able to gather information about your recalls and the notifications sent for those recalls. You will also be able to add a GTIN/NDC to your account, assign resolvers to those GTIN/NDCs and create/edit Resolver Links tied to those resolvers.
As a Distributor or Dispenser, you will be able to gather the notifications sent to you and acknowledge those recall notifications.
As a Third Party, you will be able to do the above for the appropriate type of account that you are responsible for.
Overview of the API
The API uses token based authentication.
Other forms of authentication, ATP for example, may be added later and this document will be updated accordingly.
This document will show all API calls with examples in both Python and CURL. The Python version is expected to use the latest version of the Python library.
Using the API
Authentication
The Trust.med API uses Token based Authentication. In order to use the API, you will need to be provided with a username and password by your Trust.med Representative.
Sandbox Testing
In order to test the Trust.med API within a Sandbox environment, there are a few extra steps:
You will need to have your Trust.med representative provision a TESTING API username and password for the system. This is a different set of credentials than those mentioned in the “Before You Begin” section.
You will need to provide the IP addresses for all of the test machines to your Trust.med representative.
All endpoints in this document will need to have the “https://atp.med” replaced with “https://staging.atp.med”
Capabilities
Once you have credentials and are able to access the system you will be able to do the following:
Need Assistance?
Endpoints
Generating a Token
POST /token
The Trust.med API uses the Authorization token sent in the Header of a request to authenticate a user.
Example Body: POST
The header will look like the following:
"Authorization: Bearer 123abc...987zyx"
In order to generate the access token, follow these steps:
1. Gather your username and password.
2. Send a request to the Access Token Generation endpoint.
3. You will send the username, password, scope, grant_type and client_id as Post Body elements.
Example Body:
{
"client_id": "dotmed",
"grant_type": "password",
"scope": "openid",
"username": "your-username",
"password": "your-password",
}
import requests
payload = {
'client_id': 'dotmed',
'grant_type': 'password',
'scope': 'openid',
'username': 'your-username',
'password': 'your-password',
}
r = requests.post("https://atp.med/api/v1.0/token", data=payload)
cURL:
curl --request POST \
--url 'http://api.med/api/v1.0/token' \
--header 'content-type: application/json' \
--data '{"client_id": "dotmed", "grant_type": "password", "scope": "openid", "username": "your-username", "password": "your-password"}'
List Manufacturer Recalls
This endpoint provides a list of active recalls.
Manufacturers engaging with this endpoint will receive a list of their active recalls.
Dispensers and Wholesalers engaging with this endpoint will receive a list of active recalls initiated by manufacturers.
In order to use this endpoint, you will need to Generate an Access Token first. Once you have your access token, you will attach the access token to the header as a "Bearer Token" authorization type.
This endpoint will return a list of recall
objects. Each will have the following schema:
Name | Type | Description |
---|---|---|
id | number | .Med ID for the recall |
identifier | String | Recall submitter’s identifier for recall |
manufacturer | String | Manufacturer’s name |
manufacturer_event_number | String | Manufacturer event number/id |
manufacturer_phone | String | Phone number including country code |
initiation_date | Date | Date the recall was initiated |
root_cause | String | The location of the root cause pdf |
reason | String | The reason for this recall |
recall_level | Number | The level of the recall 1 - Wholesale, 2 - Dispenser, 3 - Patient |
recall_class | Number | Recall's Class (1, 2, or 3) |
stop_immediately | Boolean | True / False |
letter_wholesale | String | Download URL of the wholesale letter pdf |
letter_pharmacy | String | Download URL of the dispenser letter pdf |
letter_patient | String | Download URL of the patient letter pdf |
products | Object | List of product objects |
products: name | String | Name of the product being recalled |
products: earliest_ship_date | Date | Earliest Ship Date for the product |
products: ndc_list | Object | List of NDC objects containing all NDC information |
products: ndc_list: inner_label | String | Inner Item NDC |
products: ndc_list: outer_label | String | Carton NDC |
products: ndc_list: distributor | Array | List of distributors for the product |
products: ndc_list: lots | Object | Lots associated with the NDC |
products: ndc_list: lots: number | String | Lot Number |
products: ndc_list: lots: expiration_date | Date | Expiration date for the lot |
products: ndc_list: lots: sgtin | Array | List of SGTINs associated with the lot |
company | Number | The .Med Id for the company initiating the recall |
created_by | Number | The .Med Id for the user initiating the recall |
creator_email | String | The email of the user initiating the recall |
Accepted Method(s): GET
Examples
Python
For this example, first make sure the requests
library is installed.
python -m pip install requests
token = getAccessToken() # Defined by GenerateAccessToken endpoint
url = 'https://atp.med/api/v1.0/recall/'
headers = {'Authorization': f'Bearer {token}'}
r = requests.get(url, headers=headers)
print(r.json())
cURL
curl --request GET
--url 'https://atp.med/api/v1.0/recall/'
--header 'Authorization: Bearer abc123...x_yz'
Response
[
{
"id": 12345,
"identifier": "...",
...
"products": [
{
"id": 123,
...,
"ndc_list": [
{
"id": 4523,
"inner_label": "12345-123-01",
...
},
...
]
},
...
]
},
...
]
List Notifications for a Recall
After obtaining a list of the manufacturer recalls (List Manufacturer Recalls endpoint), you can extract a specific Recall ID from the response. This endpoint is used to generate a list of all of the notifications distributed for a particular recall.
In order to use this endpoint, you will need to Generate an Access Token first. Once you have your access token, you will attach the access token to the header as a “Bearer Token” authorization type.
This endpoint will return a list of Notification
objects, each will have the following schema:
Name | Type | Description |
---|---|---|
id | Number | .Med ID for the notification |
recall_id | Number | .Med ID for the recall |
acknowledge | Boolean | Whether the notification has been acknowledged |
ack_date | DateTime | Date of the acknowledgment |
created_at | DateTime | When the notification was created |
last_notified | DateTime | When the notification was last delivered |
company | Object | Details about the company the notification was sent to |
company: name | String | The company's name |
company: type | Number | The type of company 2 - Dispenser, 3 - Distributor |
location | Object | Details about the individual location the data was sent |
location:store_identifier | String | Identifier or Name of the Store |
location:address,city,state,zip | String | Address information for the location |
Accepted Method(s): GET
Examples
Python
For this example, first make sure the requests
library is installed.
python -m pip install requests
import requests
token = getAccessToken() # Defined by GenerateAccessToken endpoint
company_id = 123
url = f'https://atp.med/api/v1.0/recall/{recall_id}/notification/'
headers = {'Authorization': f'Bearer {token}'}
r = requests.get(url, headers=headers)
print(r.json())
cURL
curl --request GET
--url 'https://atp.med/api/v1.0/recall/:recall_id/notification/'
--header 'Authorization: Bearer abc123...x_yz'
Response
[
{
"id": 12345,
"acknowledge": true,
...
"company": {...},
"location": {...},
"recall": {...},
},
...
]
Gathering New Recall Notifications (Auto-Acknowledged)
This endpoint will be utilized to gather all new Recall Notifications tied to the locations that the user manages. This endpoint returns data in the same fashion as the Recall endpoint, but it ensures that the data returned is only new non-accessed Notifications.
This endpoint is different from the recall or notifications endpoint in that it is a mixture of both plus a filter to only provide new information, thus providing API partners with a way to do both actions within a single call for the freshest data.
In order to use this endpoint, you will need to Generate an Access Token first. Once you have your access token, you will attach the access token to the header as a “Bearer Token” authorization type.
Accepted Method(s): GET
Examples
Python
For this example, first make sure the requests
library is installed.
python -m pip install requests
import requests
token = getAccessToken() # Defined by GenerateAccessToken endpoint
url = f'https://atp.med/api/v1.0/recall-notifications/'
headers = {'Authorization': f'Bearer {token}'}
r = requests.get(url, headers=headers)
print(r.json())
cURL
curl --request GET
--url 'https://atp.med/api/v1.0/recall-notifications/'
--header 'Authorization: Bearer abc123...x_yz'
Response
[
{
"id": 12345,
"products": [{
"...",
"ndc_list": [{
"outer_ndc": "",
...
},
...]
}, ...]
},
]
Example using Postman
List Notifications for a Recall
1. Set postmans URL to a “POST” type via the drop down and type in the endpoint:
https://atp.med/api/v1.0/token
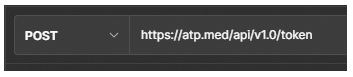
2. Select “Body”, change the type to “raw” and the format to "JSON".
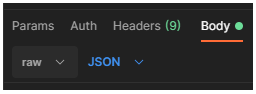
3. Within the body, put the following values:
"client_id": "dotmed",
"grant_type": "password",
"scope": "openid",
"username": (Your .Med Provided API Username),
"password": (Your .Med Provided API Password)
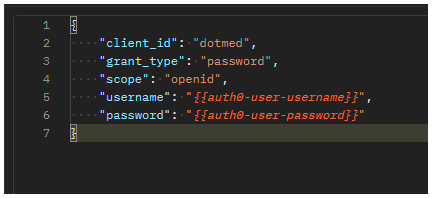
4. Extract the token value from the response.
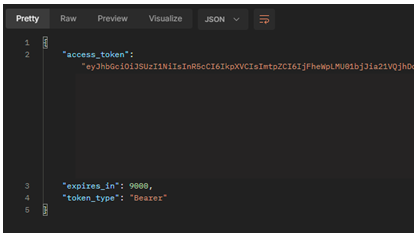
Need Help? Schedule a Meeting.
Talk with a member of our technical team and get your API questions answered today!